On this article, we’ll proceed our Java design patterns research by trying on the Proxy Design Sample. It is without doubt one of the Structural Design Patterns, and we will discover it in virtually in JDK and Spring frameworks. Utilizing a Java software, we’ll work out what this sample is all about. After that, we’ll take a look at the sample’s design benefits, utilization, and drawbacks.
The Proxy Design Sample is a structural design sample that allows us to create a stand-in or alternative for one more object. Utilizing a proxy, it’s possible you’ll conduct an motion both earlier than or after the request reaches the unique object, controlling entry to it.
Why would you wish to limit who has entry to one thing? As an illustration, think about that you’ve a big merchandise that makes use of plenty of system assets. You don’t all the time want it, however typically you do. One option to remedy it by utilizing lazy initialization, which might let you construct an object solely when one is required. Some postponed initialization code must be executed by every shopper of the thing. Sadly, there would doubtless be an excessive amount of code duplication.
Utilizing the proxy design sample, develop a brand new proxy class that has the identical interface as a main service object. You then replace your app to cross the proxy object to all of the purchasers of the unique object. The proxy builds an precise service object and transfers all duty to it after receiving a request from a shopper.
It’s all the time higher to grasp Java design sample utilizing an instance. Let’s take the instance of web entry to the worker to grasp the proxy sample extra clearly. We’ll undergo the distinct steps to make clear the design sample.
Step 1: First, let’s create an interface
Step 2: To grant the authorization to the actual worker, create an
Step 3: To supply the thing of the EmployeeInternetAccess class, create a
Step 4: Create a category referred to as
Step 5: Output
Let’s take a look at the category diagram for a greater understanding
Proxy Design Sample
Let’s take a look at a few of the actual work instance of the Proxy Design Sample.
Earlier than utilizing any design sample, it’s all the time good to finds of the benefits and drawbacks of the design sample.
The most important distinction between the 2 patterns is the burdens every sample carries. Decorators focus on including duties, whereas proxies focus on limiting entry to an object.
On this submit, we talked in regards to the Proxy Design Sample. We noticed a few of the real-world examples together with what are some benefits of utilizing this sample. We additionally noticed a Java implementation for this design sample. You may all the time verify our GitHub repository for the newest supply code.
Proxy Design Sample
The Proxy Design Sample is a structural design sample that allows us to create a stand-in or alternative for one more object. Utilizing a proxy, it’s possible you’ll conduct an motion both earlier than or after the request reaches the unique object, controlling entry to it.
Drawback
Why would you wish to limit who has entry to one thing? As an illustration, think about that you’ve a big merchandise that makes use of plenty of system assets. You don’t all the time want it, however typically you do. One option to remedy it by utilizing lazy initialization, which might let you construct an object solely when one is required. Some postponed initialization code must be executed by every shopper of the thing. Sadly, there would doubtless be an excessive amount of code duplication.
Answer
Utilizing the proxy design sample, develop a brand new proxy class that has the identical interface as a main service object. You then replace your app to cross the proxy object to all of the purchasers of the unique object. The proxy builds an precise service object and transfers all duty to it after receiving a request from a shopper.
1. Proxy Design Sample Java
It’s all the time higher to grasp Java design sample utilizing an instance. Let’s take the instance of web entry to the worker to grasp the proxy sample extra clearly. We’ll undergo the distinct steps to make clear the design sample.
Step 1: First, let’s create an interface
InternetAccess
to offer web entry to the workers:
Code:
public interface InternetAccess {
public void grantInternetAccessToEmployees();
}
Step 2: To grant the authorization to the actual worker, create an
EmployeeInternetAccess
class that implements the InternetAccess
interface.
Code:
public class EmployeeInternetAccess implements InternetAccess {
non-public String employeeName;
@Override
public void grantInternetAccessToEmployees() {
System.out.println("Web Entry granted for worker: " + employeeName);
}
public EmployeeInternetAccess(String empName) {
this.employeeName = empName;
}
public String getEmployeeName() {
return employeeName;
}
public void setEmployeeName(String employeeName) {
this.employeeName = employeeName;
}
}
Step 3: To supply the thing of the EmployeeInternetAccess class, create a
ProxyInternetAccess
class that implements the InternetAccess
interface
Code:
public class ProxyEmployeeInternetAccess implements InternetAccess {
non-public String employeeName;
non-public EmployeeInternetAccess employeeInternetAccess;
public ProxyEmployeeInternetAccess(String employeeName) {
this.employeeName = employeeName;
}
@Override
public void grantInternetAccessToEmployees() {
if (getRole(employeeName) > 4) {
employeeInternetAccess = new EmployeeInternetAccess(employeeName);
employeeInternetAccess.grantInternetAccessToEmployees();
} else {
System.out.println("No Web entry granted. Your job degree is beneath 5");
}
}
public int getRole(String empName) {
//make a DB name to get the worker position and return it.
return 31;
}
}
Step 4: Create a category referred to as
ProxyPatternClient
that may present staff the entry to the web.
Code:
public class ProxyPatternClient {
public static remaining String EMPLOYEE_NAME = "Aayush Sharma";
public static void major(String[] args) {
InternetAccess internetAccess = new ProxyEmployeeInternetAccess(EMPLOYEE_NAME);
internetAccess.grantInternetAccessToEmployees();
}
}
Step 5: Output
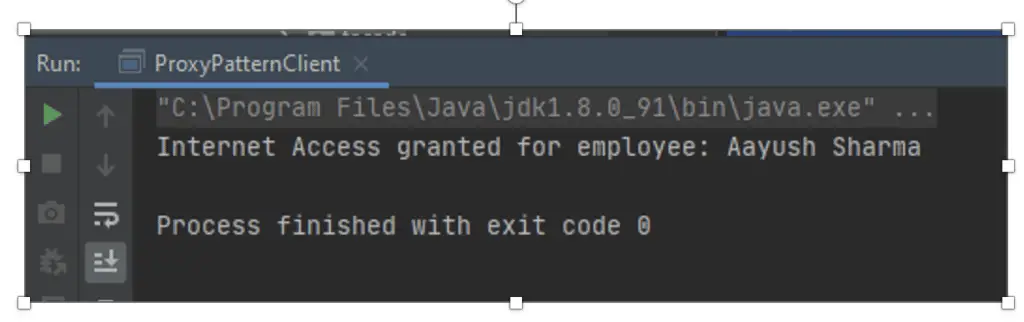
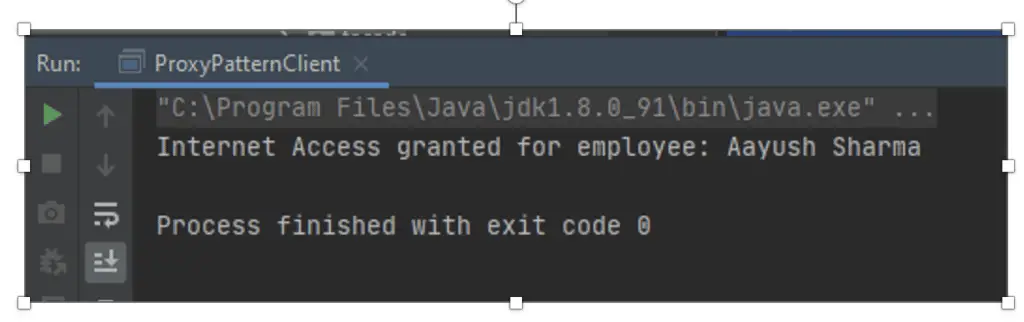
2. Class Diagram
Let’s take a look at the category diagram for a greater understanding
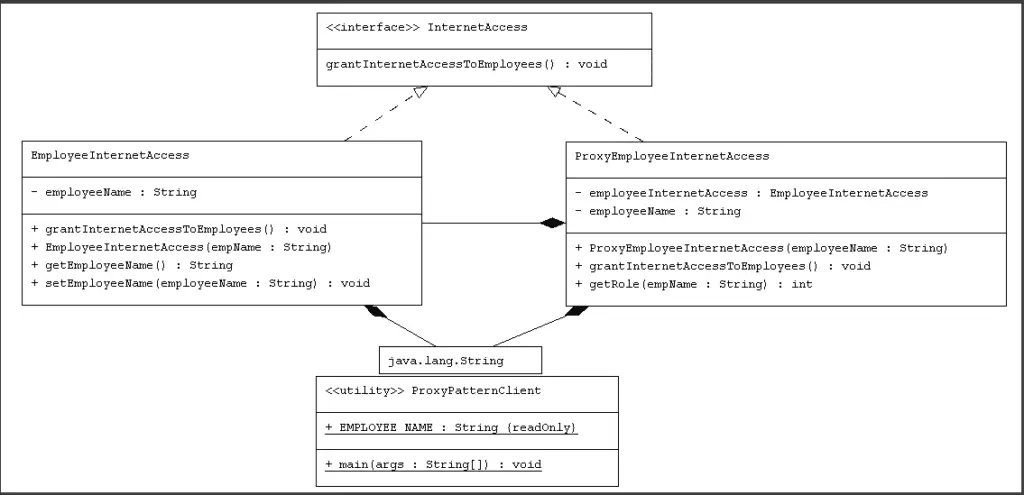
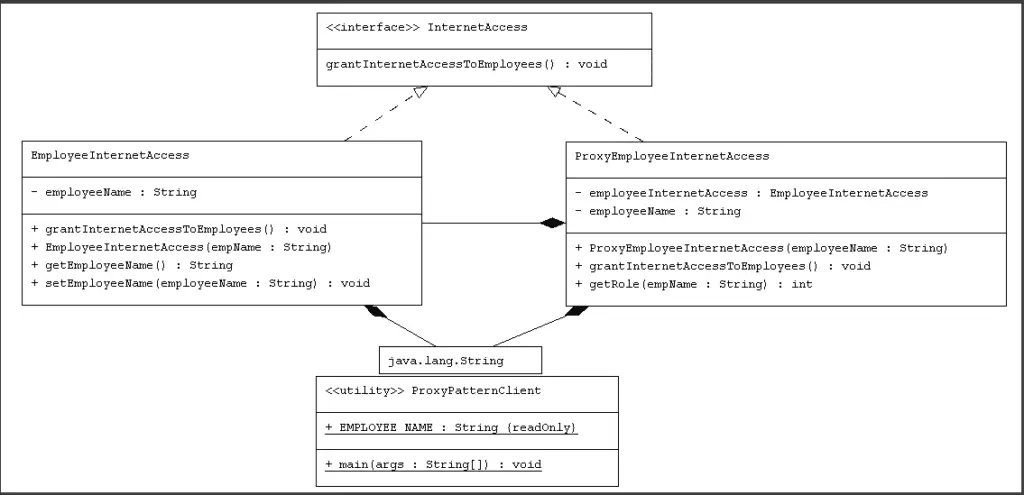
Proxy Design Sample
2.1. Actual-World Examples
Let’s take a look at a few of the actual work instance of the Proxy Design Sample.
- In hibernate, we create the code to retrieve entities from the database. Hibernate returns an object that serves as a proxy for the underlying entity class. It does this by dynamically extending the area class. The shopper software program can use the proxy to learn any knowledge it requires. With the usage of these proxy entity courses, we will implement lazy loading situations during which it solely fetched related entities when they’re particularly required. It aids in enhancing the effectiveness of DAO actions.
- It protected web entry by way of a community proxy on enterprise networks. All community queries undergo a proxy, which checks them for requests from domains which can be authorised earlier than posting the info to the community. If a request seems suspicious, the proxy ought to cease it; in any other case, the request ought to undergo.
- An object produced by the AOP framework in aspect-oriented programming (AOP) to hold out the facet contracts (advise technique executions and so forth). For example, a JDK dynamic proxy or a
CGLIB
proxy could be anAOP
proxy within theSpring AOP
.
2.2. Completely different Proxies
- Distant proxy -> depicts a distantly lactated object. The shopper should put extra effort into community connection in the event that they wish to talk with distant objects. Purchasers focus on having real conversations whereas a proxy object handles this communication on the unique object’s behalf.
- Digital proxy -> delays the on-demand technology and initialization of pricy objects earlier than they’re wanted. Digital proxies embrace entities produced by Hibernate.
- Safety proxy -> helps put in place safety over the unique object. Earlier than calling a way, they could verify the entry privileges after which grant or refuse entry in accordance with the outcomes.
- Sensible Proxy -> conducts further clerical work each time a shopper accesses an object. An illustration could be to verify that it locked the precise object earlier than accessing it to make it possible for no different object could alter it.
3. Benefits and Disadvantages of Proxy Sample
Earlier than utilizing any design sample, it’s all the time good to finds of the benefits and drawbacks of the design sample.
3.1. Benefits
- Safety is one advantage of the proxy sample.
- This design prevents the duplication of doubtless enormously massive and memory-intensive gadgets. This improves the appliance’s efficiency.
- By putting in an area code proxy (stub) on the shopper laptop after which connecting to the server utilizing distant code, the distant proxy additionally assures safety.
3.2. Disadvantages
- This sample provides one other layer of abstraction, which may sometimes trigger issues if some purchasers entry the Actual topic code straight whereas others may entry the Proxy courses. This might trigger inconsistent habits.
4. When to Use Proxy Design Sample?
- To supply a surrogate or placeholder for one more object to manage entry to it.
- To help distributed, regulated, or clever entry, add one other indirection.
- To forestall the actual element from changing into overly advanced, add a wrapper and delegation.
4.1. Proxy vs Decorator Sample
The most important distinction between the 2 patterns is the burdens every sample carries. Decorators focus on including duties, whereas proxies focus on limiting entry to an object.
Abstract
On this submit, we talked in regards to the Proxy Design Sample. We noticed a few of the real-world examples together with what are some benefits of utilizing this sample. We additionally noticed a Java implementation for this design sample. You may all the time verify our GitHub repository for the newest supply code.